入力内容が正しく入力されているかチェックし、エラーがあればErrorProviderを使ってエラーの内容を通知するサンプルです。
ErrorProviderにエラーのあったコントロールと警告のメッセージを渡すと、コントロール横に警告を示すICONが表示されます。また、そのICONにカーソルをあてると警告のメッセージが表示されます。
こんな感じです。 エラーの内容をまとめて通知可能です。
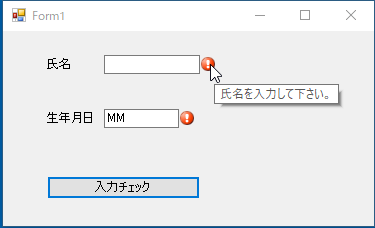
サンプルの内容
① ErrorProviderをクリアする。
② 氏名の未入力チェックをする。
未入力ならErrorProviderにエラーを通知する。
③ 生年月日の日付チェックをする。
日付でなければErrorProviderにエラーを通知する。
指定可能なICONの点灯スタイル
BlinkStyleは3つの点灯スタイルを指定できます。
AlwaysBlink | 常に点滅 |
BlinkIfDifferentError | 始めに点灯し、時間がたつと点灯しなくなる |
NeverBlink | 点滅しない |
使用部品
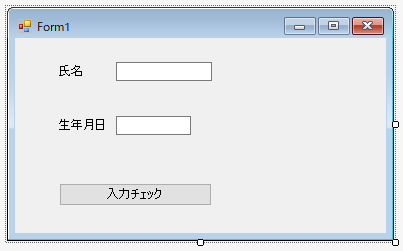
No | 項目名 | Text | name | 部品 |
1 | 氏名(見出し) | 氏名 | label1 | Label |
2 | 氏名(入力欄) | 空 | txtFullName | TextBox |
3 | 生年月日(見出し) | 生年月日 | label2 | Label |
4 | 生年月日(入力欄) | 空 | txtBirthday | TextBox |
5 | 入力チェックボタン | 入力チェック | btnCheck | Button |
プログラミング
言語:C#
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp17
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
// ErrorProviderのインスタンスを生成
ErrorProvider errorProvider = new ErrorProvider();
/// <summary>
/// 画面起動時処理
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void Form1_Load(object sender, EventArgs e)
{
// アイコンを点滅なしに設定する
errorProvider.BlinkStyle = ErrorBlinkStyle.NeverBlink;
}
/// <summary>
/// チェックボタンクリック処理
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void btnCheck_Click(object sender, EventArgs e)
{
// ErrorProviderをクリアします。
errorProvider.Clear();
string msg = "";
// 氏名の未入力チェックをする。
if (String.IsNullOrEmpty(txtFullName.Text))
{
// 未入力ならエラーを通知する。
msg = "氏名を入力して下さい。";
errorProvider.SetError(txtFullName, msg);
}
// 生年月日の日付チェックをする。
DateTime dateTime;
if (!DateTime.TryParse(txtBirthday.Text, out dateTime))
{
// 日付でなければエラーを通知する
msg = "生年月日は日付で入力して下さい。";
errorProvider.SetError(txtBirthday, msg);
}
}
}
}
実行結果
生年月日に日付でない文字を入力し、入力チェックをクリックする。
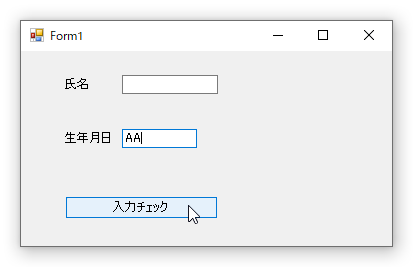
氏名と生年月日にエラーICONが表示される。
氏名のエラーを確認する。
氏名を入力し、入力チェックをクリックする。
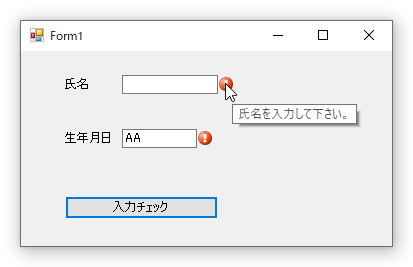
氏名のエラーが消える。
生年月日のエラーを確認する。
生年月日を入力し、入力チェックをクリックする。
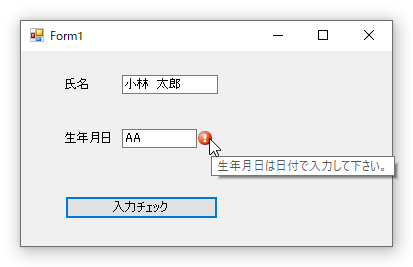
生年月日のエラーが消える。
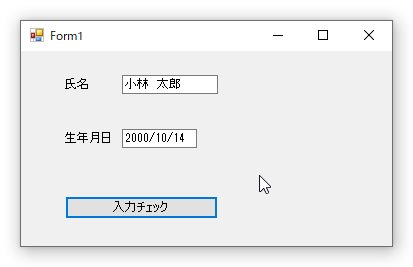
以上です。